Inputs
The input or source determines and controls the origin of the audio data. We have multiple available inputs, and each one can manage different audio feeding method. For example, to use audio files we are going to need the Player or the Extractor Object. For a microphone we have the Capturer or the OVR, depends on the target platform. We have too the ability to listen the audio from other devices using the Loopback input (only works on Windows platforms for now), and with the Stream Object we can inject directly the audio data from other sources.
Player Input
Play/Stop/Pause controls
Load audio files from the content folder
Allow seek playback
Allow adjust playback volume
Access to audio metadata
Initialization
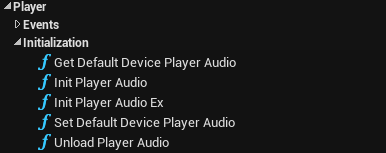
Warning
If you experience audio gliches on Android platform the buffer size must be increased. The optimal value may vary between devices.
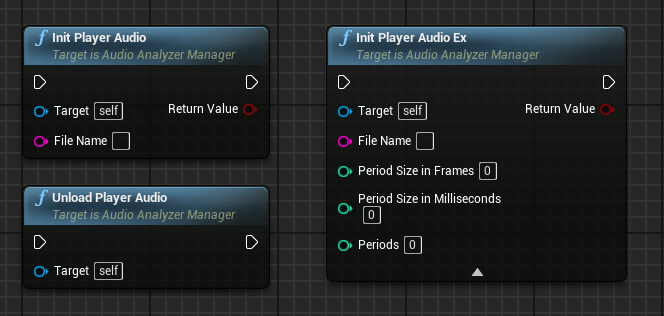
File Name |
Path to the audio file |
File Name |
Path to the audio file |
Period Size in Frames |
The desired size of a period in PCM frames (power of 2), 0 to use default value |
Period Size in Milliseconds |
The desired size of a period in milliseconds, 0 to use default value |
Periods |
The number of periods making up the device’s entire buffer, 0 to use default value. Total buffer is Period_Size * Periods |
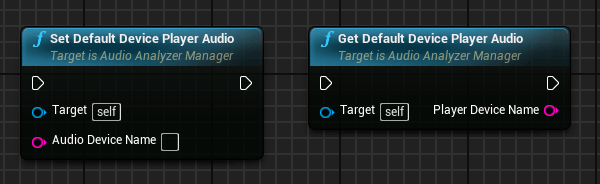
Audio Device Name |
Audio device name (From Get Output Audio Device node output) |
Asynchronous Initialization
Note
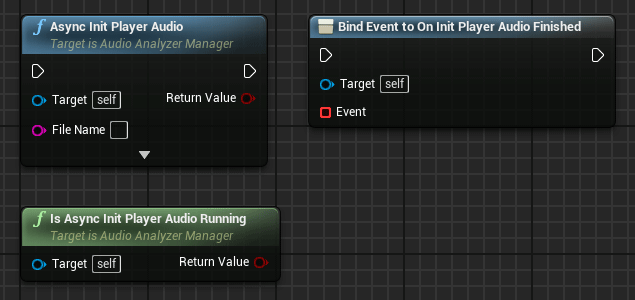
File Name |
Path to the audio file |
Period Size in Frames |
The desired size of a period in PCM frames (power of 2), 0 to use default value |
Period Size in Milliseconds |
The desired size of a period in milliseconds, 0 to use default value |
Periods |
The number of periods making up the device’s entire buffer, 0 to use default value. Total buffer is Period_Size * Periods |
Players controls
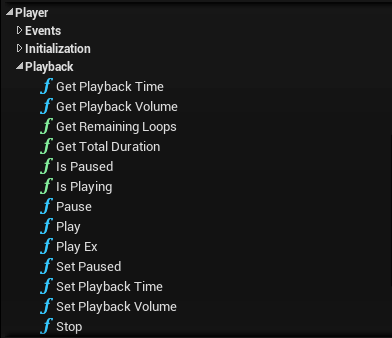
After the player initialization we can use the player nodes to control the sound playback, We can start or stop the playback, change the volume, set the playback position…
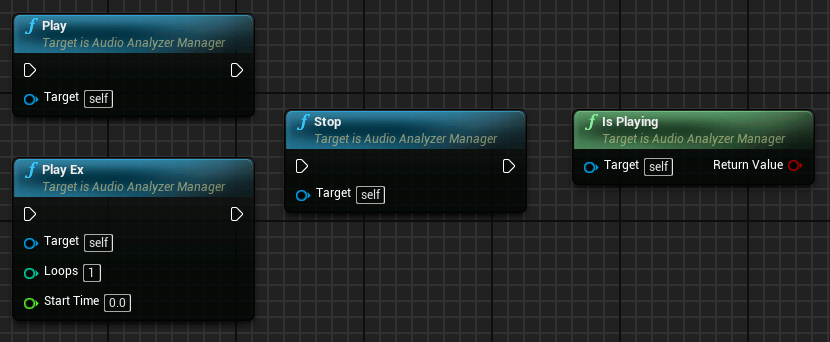
Loops |
Number of loops (0-infinite playback) |
StartTime |
Time in seconds to start the playback |
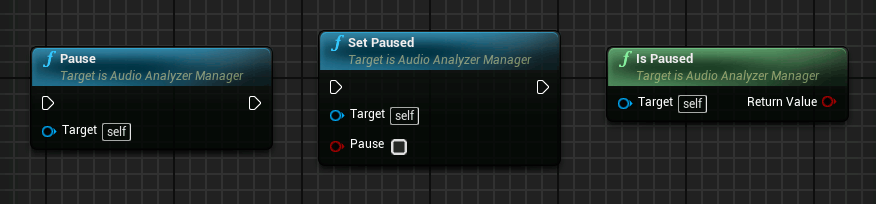
Pause |
New pause state |
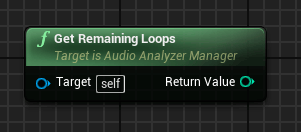
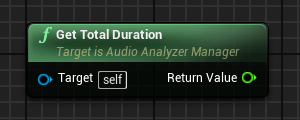
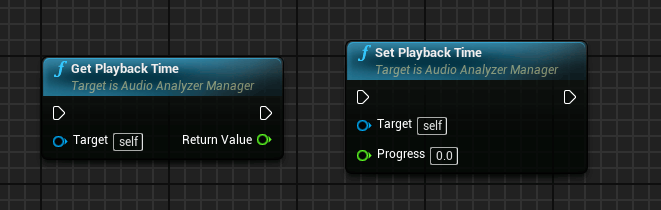
Progress |
New playback position in seconds |
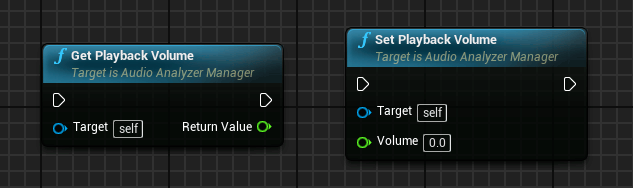
Volume |
New volume in range 0.0 - 1.0 |
Player Events
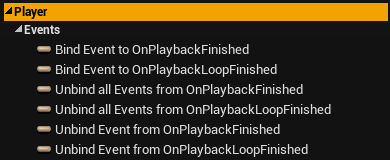
We can track some event related with the audio playback, like the end of a loop or the total file playback end.
Bindable events
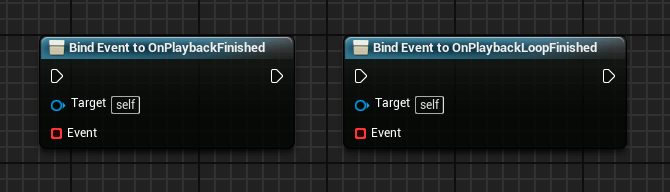
Player Utils
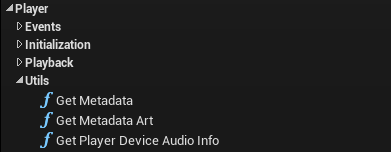
Some player extra functions
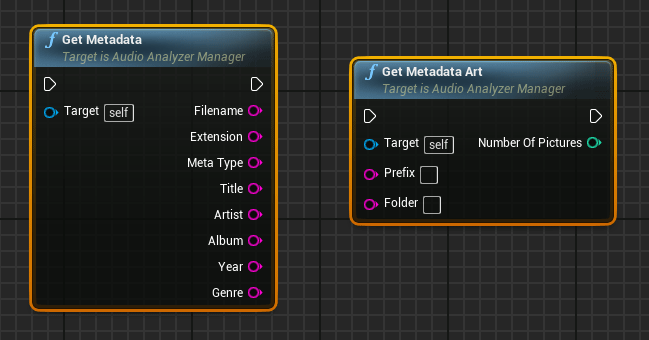
Filename |
Filename of the audio file |
Extension |
Extension of the audio file |
MetaType |
ID3_V1 , ID3_V2.3 , ID3_V2.4 |
Title |
Title of the song |
Artist |
Artist |
Album |
Album |
Year |
Year |
Genre |
Genre |
Prefix |
Prefix used to name the pictures. |
Folder |
Destination folder, must exists |
Number of files |
Number of stored pictures |
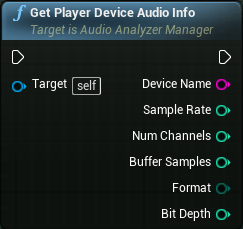
Device Name |
Name of the device in the system devices list |
Sample Rate |
Number of samples per second |
Num Channels |
Number of channels |
Buffer Samples |
Number of samples of the playback buffer |
Format |
Format of the values that represent each sample (Fixed/Float) |
Bit Depth |
Number of bits used for each sample |
Player Supported formats
This player support multiple audio formats, with wav files we are using the memory file directly so no wait time is needed, but with compressed formats a decode process is done when the audio file is loaded, so have the audio ready for the playback can take some time.
Microphone Input
Real Time capture
Volume Override
Initialization
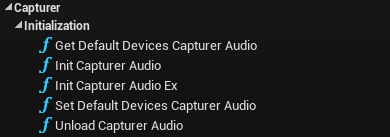
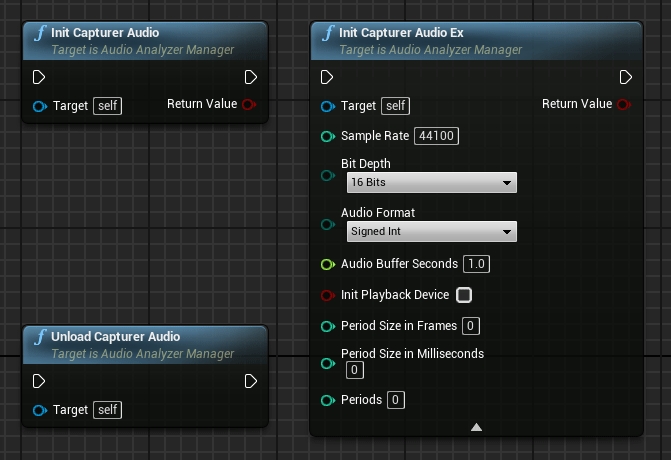
Sample Rate |
Samples per second of the capture |
Bit Depth |
Bits per sample |
Audio Format |
Sample number format (fixed/float) |
Audio Buffer Seconds |
Number of seconds of capture history |
Init Playback Device |
Initialize device to playback received data |
Period Size in Frames |
The desired size of a period in PCM frames (power of 2), 0 to use default value |
Period Size in Milliseconds |
The desired size of a period in milliseconds, 0 to use default value |
Periods |
The number of periods making up the device’s entire buffer, 0 to use default value. Total buffer is Period_Size * Periods |
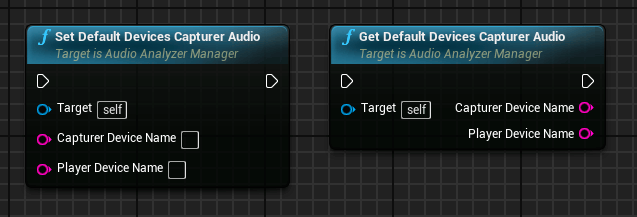
Capturer Device Name |
Audio device name to capture the sound (From Get Input Audio Device node output) |
Player Device Name |
Audio device name to play the sound (From Get Output Audio Device node output) |
Capturer Device Name |
Audio device name to capture the sound |
Player Device Name |
Audio device name to play the sound |
Capturer controls
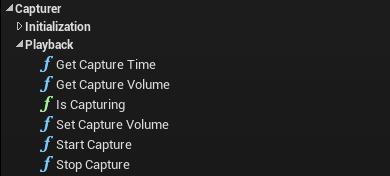
After initialization we can use the capturer nodes to control the start or end of the capture
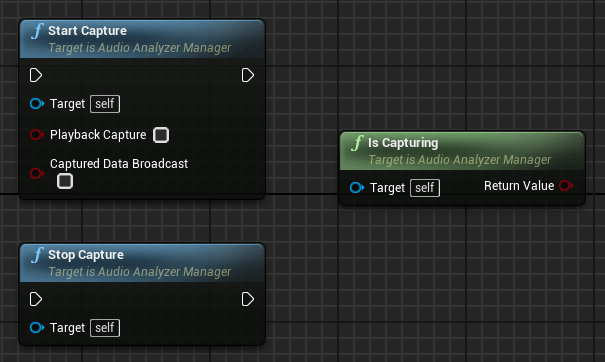
Playback Capture |
Enable to playback the capture sound at the same time, only if the playback device has been enabled in the Initialization node |
Captured Data Broadcast |
Returns the captured audio buffer using OnCapturedData Event |
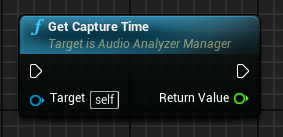
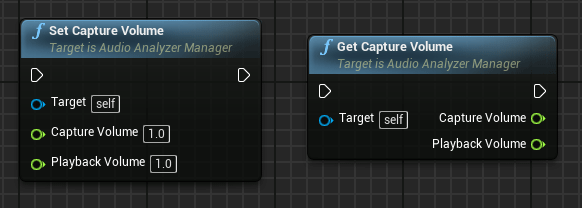
Capture Volume |
Microphone normalized volume |
Playback Volume |
Playback normalized volume |
Capture Volume |
New capture volume in range 0.0 - 1.0 |
Playback Volume |
New playback volume in range 0.0 - 1.0 |
Capturer Events
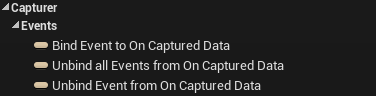
We can track some event related with audio capture. Each time a capture buffer is filled we can obtain the raw pcm audio and feed other analyzer inputs.
Bindable events
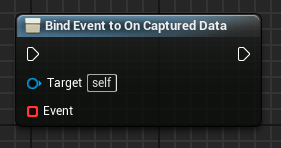
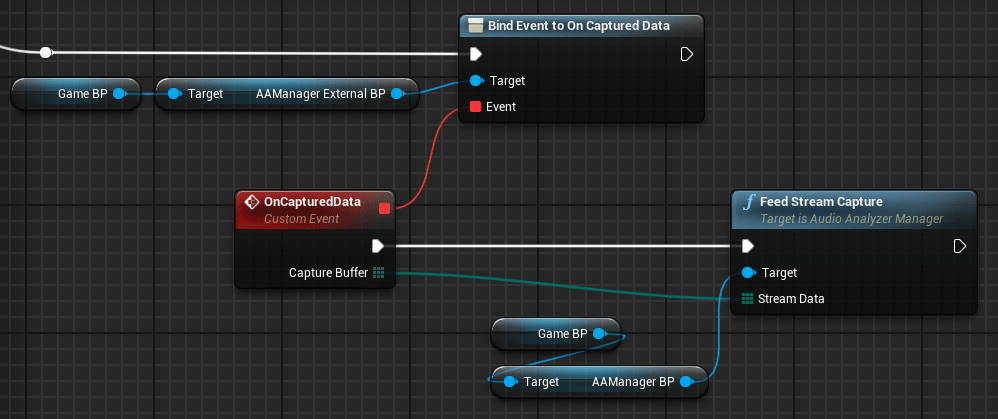
Capturer Utils

Some capturer extra functions
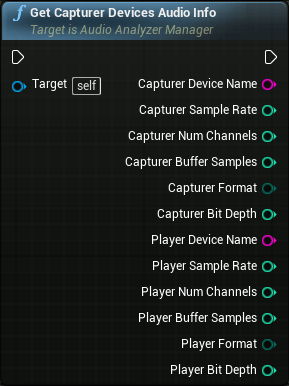
Capturer Device Name |
Name of the capturer device in the system devices list |
Capturer Sample Rate |
Number of samples per second |
Capturer Num Channels |
Number of channels |
Capturer Buffer Samples |
Number of samples of the playback buffer |
Capturer Format |
Format of the values that represent each sample (Fixed/Float) |
Capturer Bit Depth |
Number of bits used for each sample |
Player Device Name |
Name of the player device in the system devices list |
Player Sample Rate |
Number of samples per second |
Player Num Channels |
Number of channels |
Player Buffer Samples |
Number of samples of the playback buffer |
Player Format |
Format of the values that represent each sample (Fixed/Float) |
Player Bit Depth |
Number of bits used for each sample |
Loopback Input
Real Time capture
Initialization
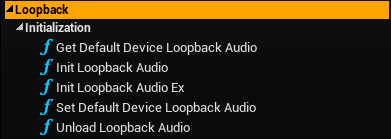
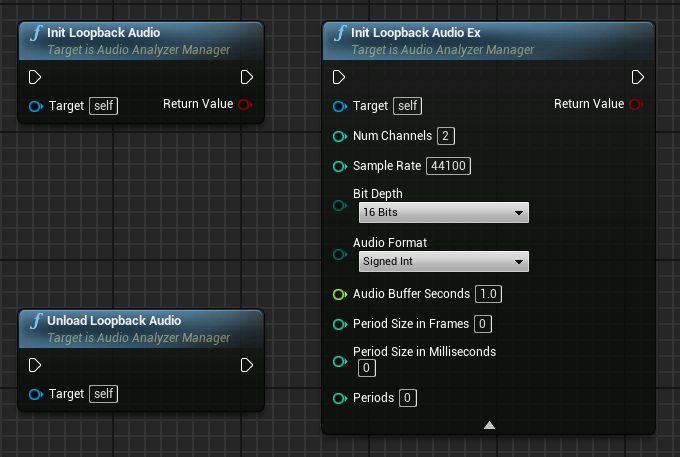
Num Channels |
Number of channels |
Sample Rate |
Number of samples per second |
Bit Depth |
Bits per sample |
Audio Format |
Sample number format |
Audio Buffer Seconds |
Number of seconds of capture history |
Period Size in Frames |
The desired size of a period in PCM frames (power of 2), 0 to use default value |
Period Size in Milliseconds |
The desired size of a period in milliseconds, 0 to use default value |
Periods |
The number of periods making up the device’s entire buffer, 0 to use default value. Total buffer is Period_Size * Periods |
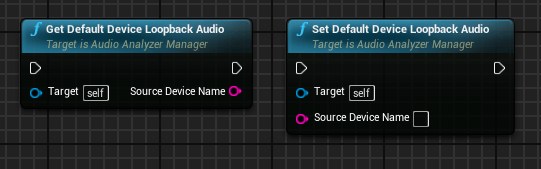
Source Device Name |
Audio device name to listen (From Get Output Audio Device node output) |
Source Device Name |
Audio device name to capture the sound |
Loopback controls
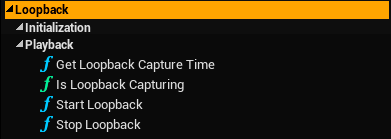
After initialization we can use the next loopback nodes to control the start/end of the capture
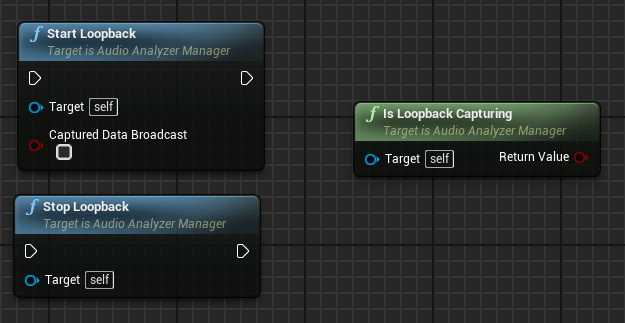
Captured Data Broadcast |
Returns the captured audio buffer using OnLoopbackCapturedData Event |
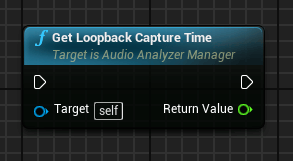
Loopback Events
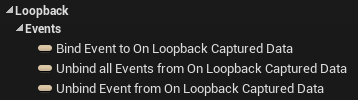
We can track some event related with audio capture. Each time a capture buffer is filled we can obtain the raw pcm audio and feed other analyzer inputs.
Bindable events
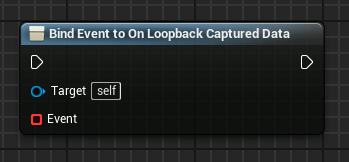
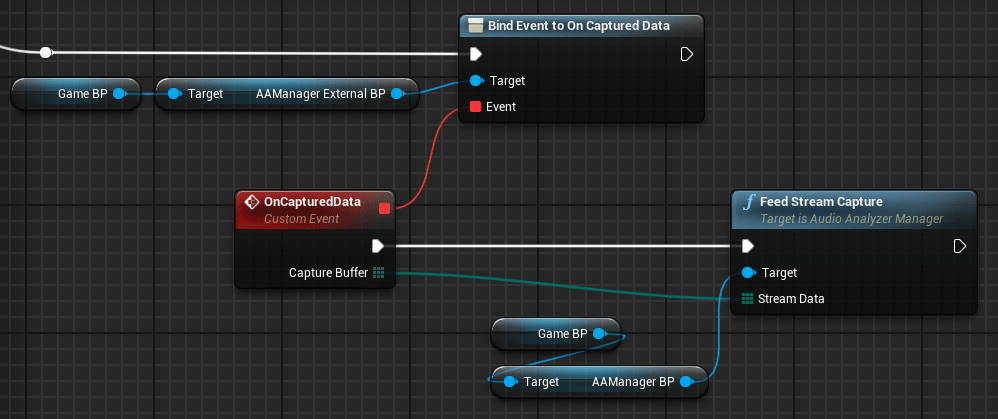
Loopback Utils
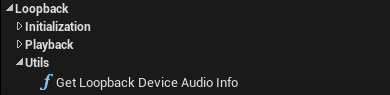
Some loopback extra functions
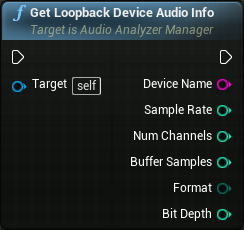
Device Name |
Name of the capturer device in the system devices list |
Sample Rate |
Number of samples per second |
Num Channels |
Number of channels |
Buffer Samples |
Number of samples of the playback buffer |
Format |
Format of the values that represent each sample (Fixed/Float) |
Bit Depth |
Number of bits used for each sample |
Extractor Input
Initialization
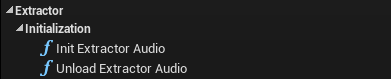
We need to uncompress the audio file before be able to do the analysis.
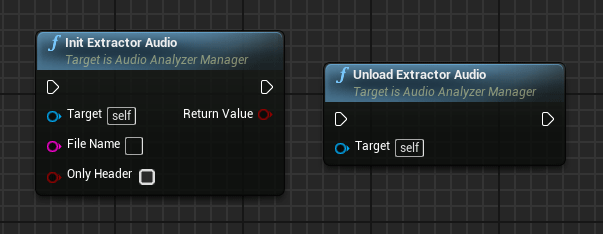
File Name |
Path to the audio file |
Only Header |
The audio data will not be uncompressed, but we can access to the metadata info |
Asynchronous Initialization
Note
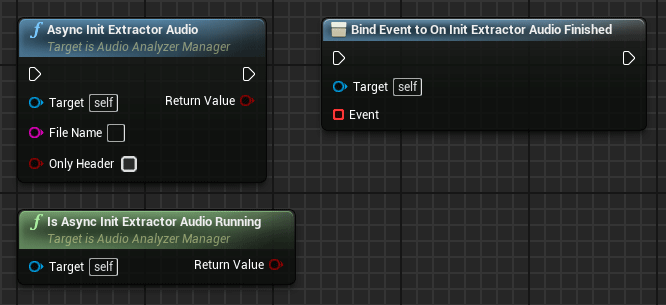
File Name |
Path to the audio file |
Only Header |
The audio data will not be uncompressed, but we can access to the metadata info |
Extractor Utils
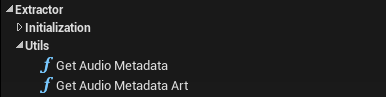
Some extractor extra functions
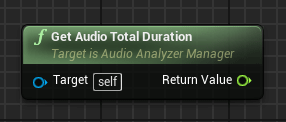
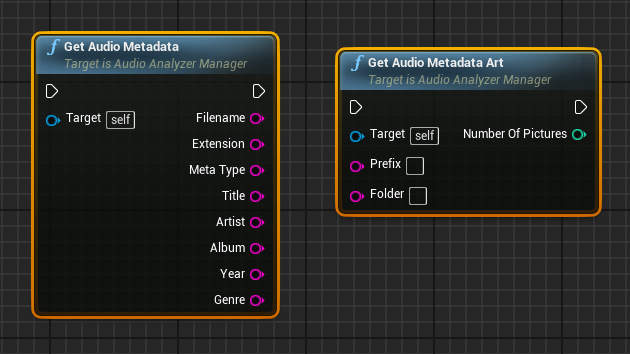
Filename |
Filename of the audio file |
Extension |
Extension of the audio file |
MetaType |
ID3_V1 , ID3_V2.3 , ID3_V2.4 |
Title |
Title of the song |
Artist |
Artist |
Album |
Album |
Year |
Year |
Genre |
Genre |
Prefix |
Prefix used to name the pictures |
Folder |
Destination folder, must exists |
Number of files |
Number of stored pictures |
Stream Input
Initialization
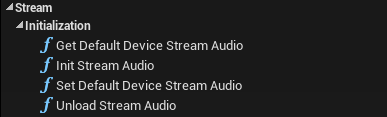
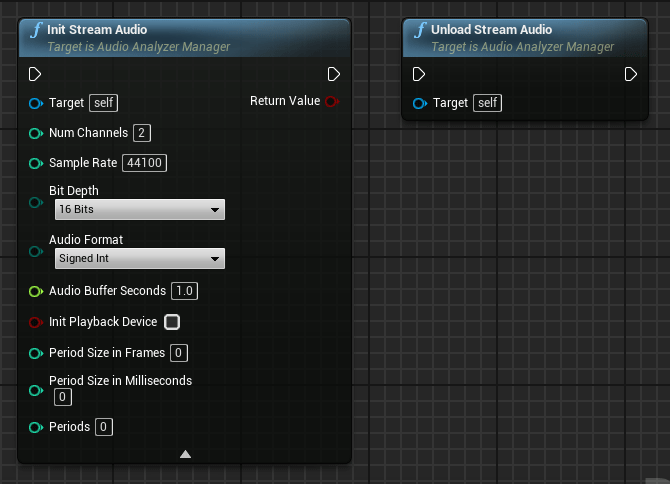
Num Channels |
Number of channels |
Sample Rate |
Samples per second |
Bit Depth |
Bits per sample |
Audio Format |
Sample number format |
Audio Buffer Seconds |
Number of seconds of capture history |
Init Playback Device |
Initialize device to playback received data |
Period Size in Frames |
The desired size of a period in PCM frames (power of 2), 0 to use default value |
Period Size in Milliseconds |
The desired size of a period in milliseconds, 0 to use default value |
Periods |
The number of periods making up the device’s entire buffer, 0 to use default value. Total buffer is Period_Size * Periods |
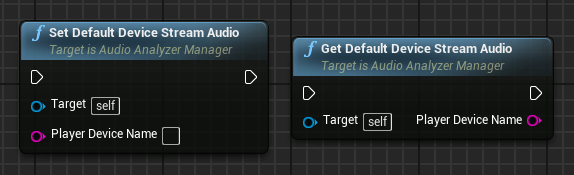
Player Device Name |
Friendly Name of audio device (GetOutputAudioDeviceNames output) |
Stream controls
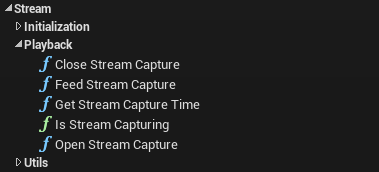
After the initialization we can feed the object using raw audio data
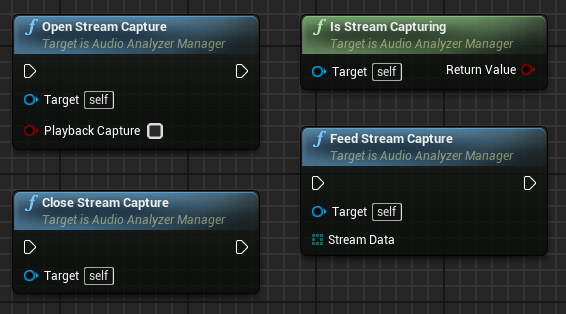
Playback Capture |
Enable to playback the capture sound at the same time, only if the playback device has been enabled in the Initialization node |
Stream Data |
TArray with the raw audio data |
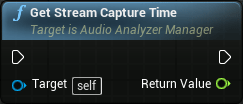
Stream Utils
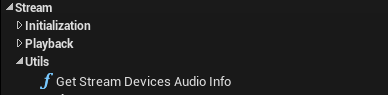
Some extractor extra functions
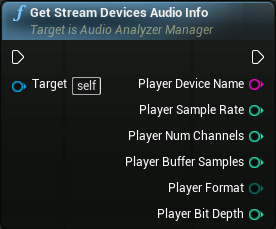
Player Device Name |
Name of the player device in the system devices list |
Player Sample Rate |
Number of samples per second |
Player Num Channels |
Number of channels |
Player Buffer Samples |
Number of samples of the playback buffer |
Player Format |
Format of the values that represent each sample (Fixed/Float) |
Player Bit Depth |
Number of bits used for each sample |
OVR Input
Initialization
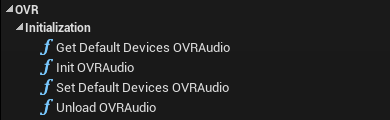
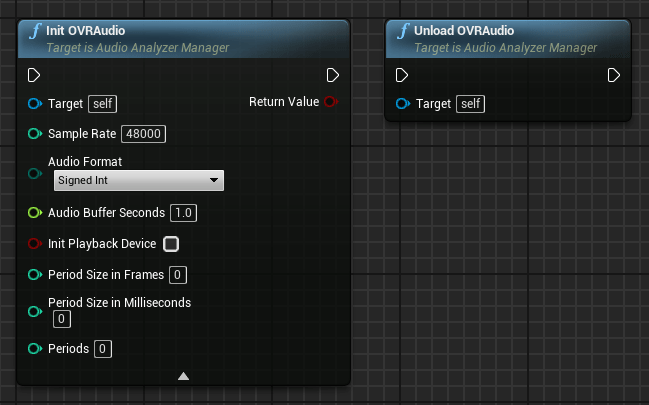
Sample Rate |
Samples per second of the capture |
Audio Format |
Sample number format (fixed/float) |
Audio Buffer Seconds |
Number of seconds of capture history |
Init Playback Device |
Initialize device to playback received data |
Period Size in Frames |
The desired size of a period in PCM frames (power of 2), 0 to use default value |
Period Size in Milliseconds |
The desired size of a period in milliseconds, 0 to use default value |
Periods |
The number of periods making up the device’s entire buffer, 0 to use default value. Total buffer is Period_Size * Periods |
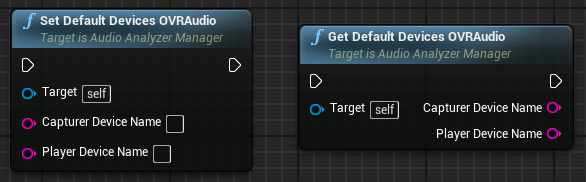
Capturer Device Name |
Audio device name to capture the sound (From Get Input Audio Device node output) |
Player Device Name |
Audio device name to play the sound (From Get Output Audio Device node output) |
Capturer Device Name |
Audio device name to capture the sound |
Player Device Name |
Audio device name to play the sound |
OVR Controls
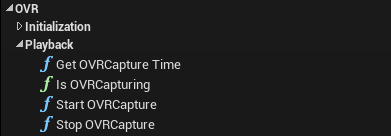
After initialization we can use this nodes to control the start or end of the capture
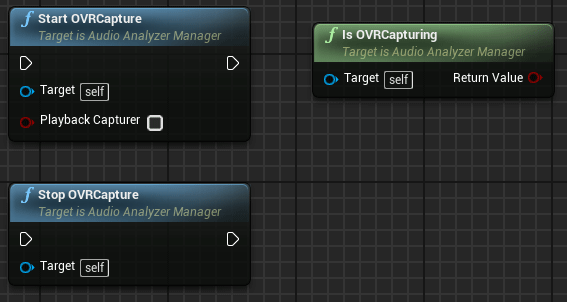
Playback Capture |
Enable to playback the capture sound at the same time, only if the playback device has been enabled in the Initialization node |
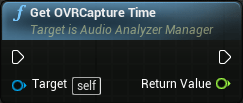
OVR Utils
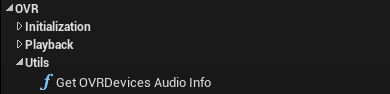
Some OVR extra functions
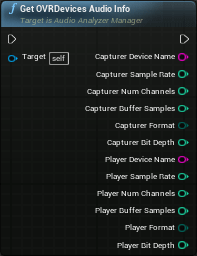
Capturer Device Name |
Name of the capturer device in the system devices list |
Capturer Sample Rate |
Number of samples per second |
Capturer Num Channels |
Number of channels |
Capturer Buffer Samples |
Number of samples of the playback buffer |
Capturer Format |
Format of the values that represent each sample (Fixed/Float) |
Capturer Bit Depth |
Number of bits used for each sample |
Player Device Name |
Name of the player device in the system devices list |
Player Sample Rate |
Number of samples per second |
Player Num Channels |
Number of channels |
Player Buffer Samples |
Number of samples of the playback buffer |
Player Format |
Format of the values that represent each sample (Fixed/Float) |
Player Bit Depth |
Number of bits used for each sample |
Soundwave Input
Initialization
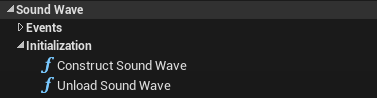
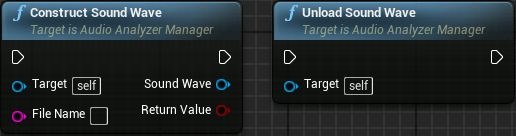
File Name |
Path to the audio file |
Asynchronous Initialization
Note
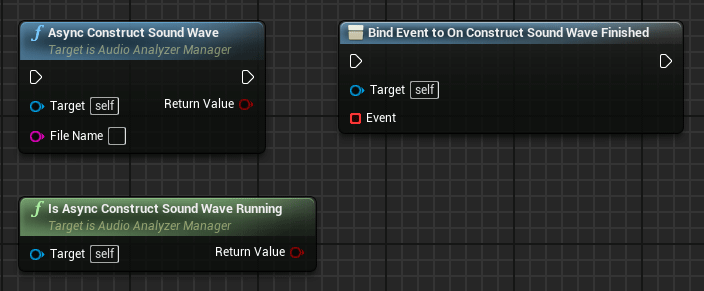
File Name |
Path to the audio file |
Soundwave playback controls
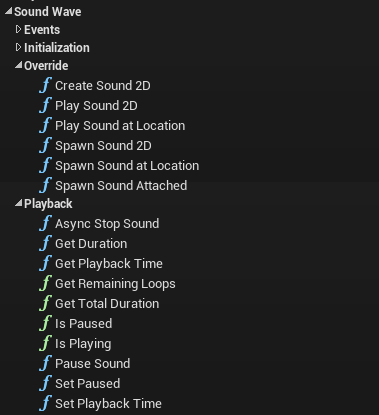
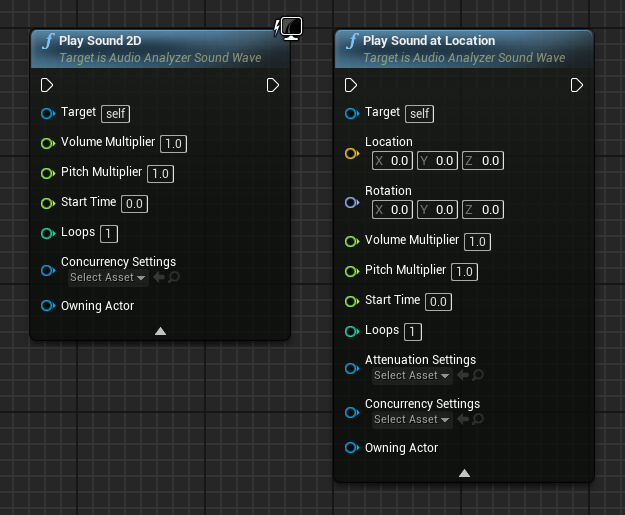
Volume Multiplier |
Multiplied with the volume to make the sound louder or softer |
Pitch Multiplier |
Multiplies the pitch |
Start Time |
How far in to the sound to begin playback at, and loop start time |
Loops |
Number of Loops (Default: 1) |
Concurrency Settings |
Override concurrency settings package to play sound with |
OwningActor |
The actor to use as the “owner” for concurrency settings purposes. Allows PlaySound calls to do a concurrency limit per owner. |
Location |
World position to play sound at |
Rotation |
World rotation to play sound at |
Volume Multiplier |
Multiplied with the volume to make the sound louder or softer |
Pitch Multiplier |
Multiplies the pitch |
Start Time |
How far in to the sound to begin playback at, and loop start time |
Loops |
Number of Loops (Default: 1) |
Attenuation_Settings |
Override attenuation settings package to play sound with |
Concurrency Settings |
Override concurrency settings package to play sound with |
OwningActor |
The actor to use as the “owner” for concurrency settings purposes. Allows PlaySound calls to do a concurrency limit per owner. |
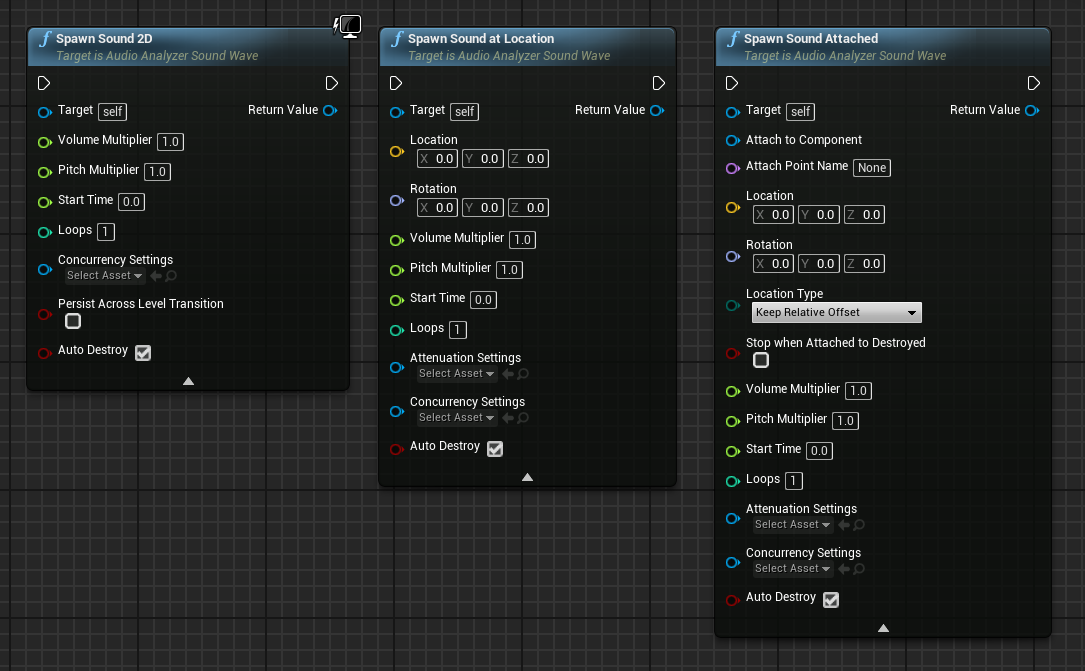
Volume Multiplier |
Multiplied with the volume to make the sound louder or softer |
Pitch Multiplier |
Multiplies the pitch |
Start Time |
How far in to the sound to begin playback at, and loop start time |
Loops |
Number of Loops (Default: 1) |
Concurrency Settings |
Override concurrency settings package to play sound with |
Persist Across Level Transition |
Whether the sound should continue to play when the map it was played in is unloaded |
Auto Destroy |
Whether the returned audio component will be automatically cleaned up when the sound finishes (by completing or stopping) or whether it can be reactivated |
Location |
World position to play sound at |
Rotation |
World rotation to play sound at |
Volume Multiplier |
Multiplied with the volume to make the sound louder or softer |
Pitch Multiplier |
Multiplies the pitch |
Start Time |
How far in to the sound to begin playback at, and loop start time |
Loops |
Number of Loops (Default: 1) |
Attenuation_Settings |
Override attenuation settings package to play sound with |
Concurrency Settings |
Override concurrency settings package to play sound with |
Auto Destroy |
Whether the returned audio component will be automatically cleaned up when the sound finishes (by completing or stopping) or whether it can be reactivated |
Attach To Component |
Component to attach to. |
Attach Point Name |
Optional named point within the AttachComponent to play the sound at |
Location |
World position to play sound at |
Rotation |
World rotation to play sound at |
Location Type |
Specifies whether Location is a relative offset or an absolute world position |
Stop When Attached To Destroyed |
Specifies whether the sound should stop playing when the owner of the attach to component is destroyed |
Volume Multiplier |
Multiplied with the volume to make the sound louder or softer |
Pitch Multiplier |
Multiplies the pitch |
Start Time |
How far in to the sound to begin playback at, and loop start time |
Loops |
Number of Loops (Default: 1) |
Attenuation_Settings |
Override attenuation settings package to play sound with |
Concurrency Settings |
Override concurrency settings package to play sound with |
Auto Destroy |
Whether the returned audio component will be automatically cleaned up when the sound finishes (by completing or stopping) or whether it can be reactivated |
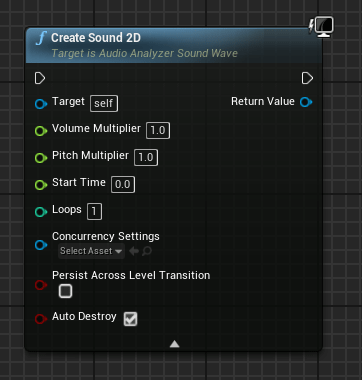
Volume Multiplier |
Multiplied with the volume to make the sound louder or softer |
Pitch Multiplier |
Multiplies the pitch |
Start Time |
How far in to the sound to begin playback at, and loop start time |
Loops |
Number of Loops (Default: 1) |
Concurrency Settings |
Override concurrency settings package to play sound with |
Persist Across Level Transition |
Whether the sound should continue to play when the map it was played in is unloaded |
Auto Destroy |
Whether the returned audio component will be automatically cleaned up when the sound finishes (by completing or stopping) or whether it can be reactivated |
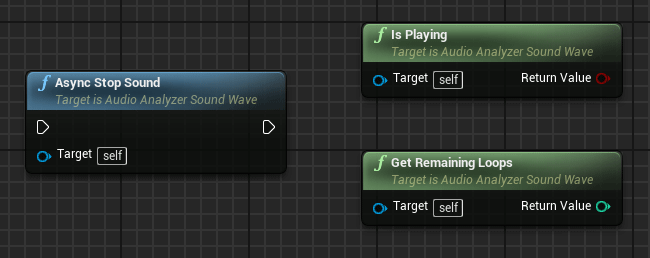
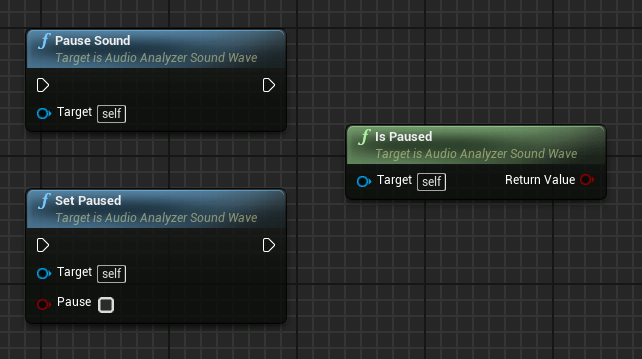
Pause |
New pause state |
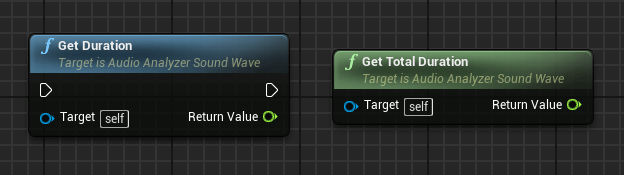
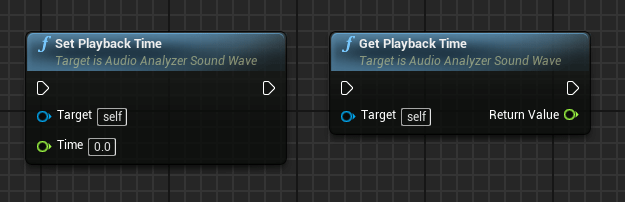
Time |
New playback position in seconds |
SoundWave Utils
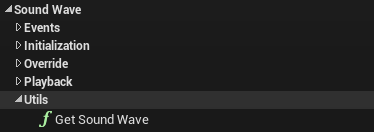
Some SoundWave related extra functions
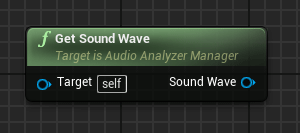
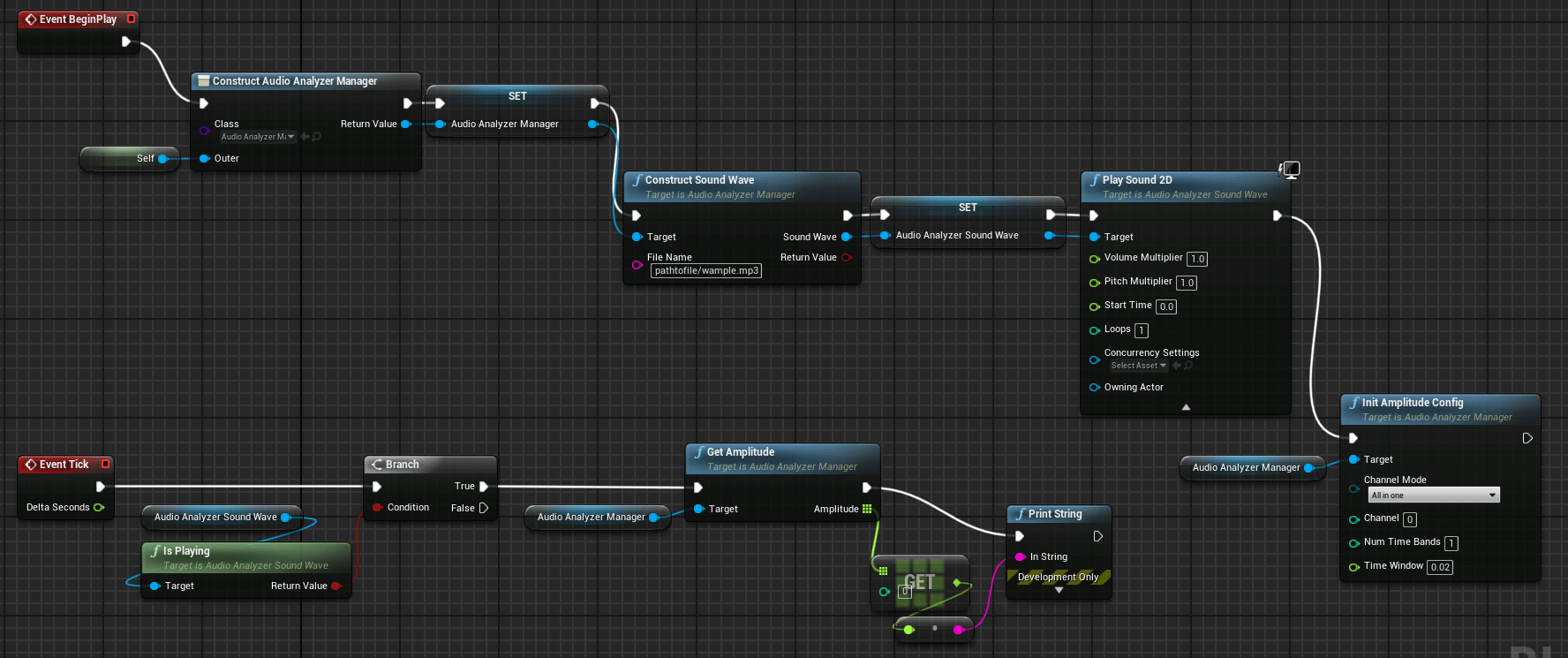
SoundWave Events
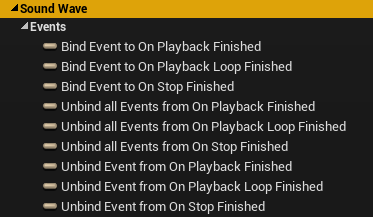
We can track some event related with the audio playback, like the end of a loop or the total file playback end.
Bindable events
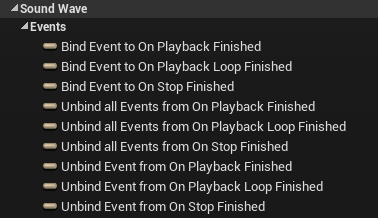
AudioComponent Input
Initialization
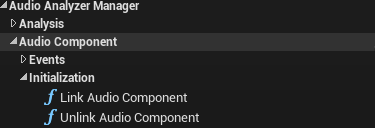
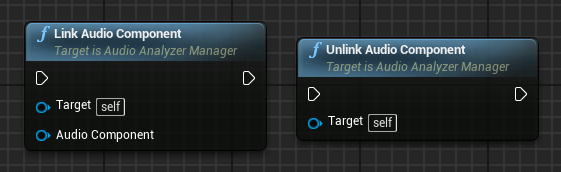
Audio Component |
Audio Component reference |
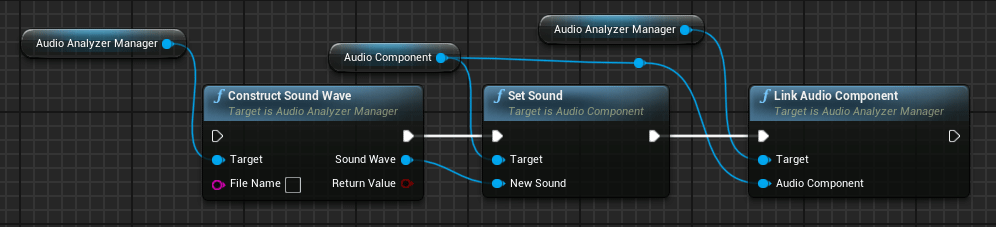
AudioComponent playback controls
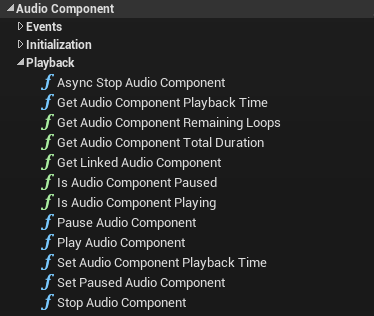
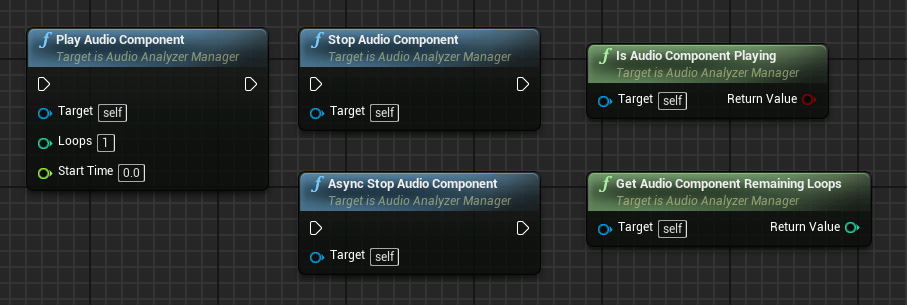
Loops |
Number of loops, (0 Infinite) |
StartTime |
Starting position in seconds |
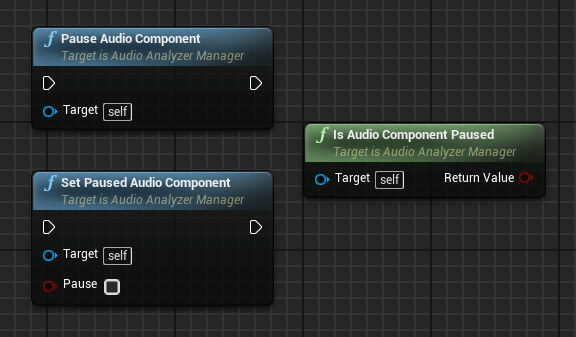
Pause |
New pause state |
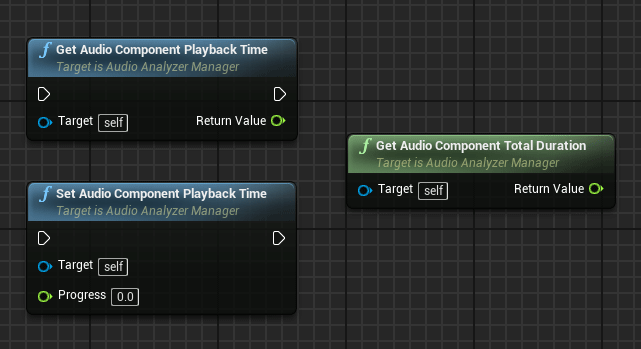
Progress |
New playback position in seconds |
AudioComponent Events
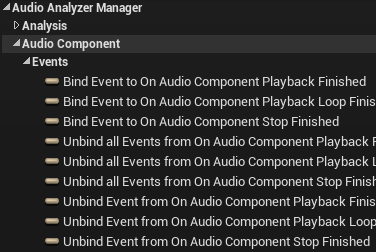
We can track some event related with the audio playback, like the end of a loop or the total file playback end.
AudioComponent Utils
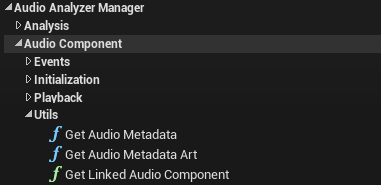
Some Audio Component related extra functions. All utils nodes of Extractor class can be used after a soundwave have been constructed.
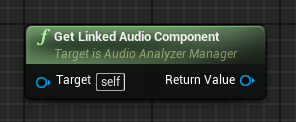
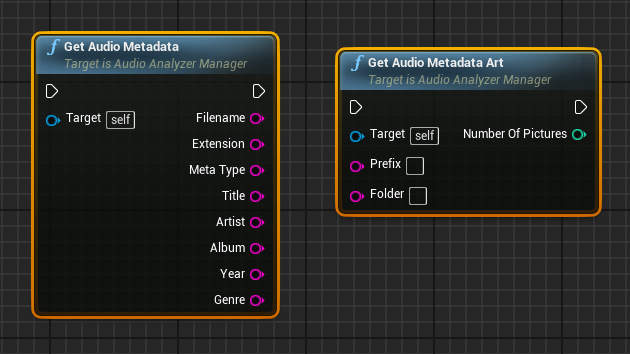
Filename |
Filename of the audio file |
Extension |
Extension of the audio file |
MetaType |
ID3_V1 , ID3_V2.3 , ID3_V2.4 |
Title |
Title of the song |
Artist |
Artist |
Album |
Album |
Year |
Year |
Genre |
Genre |
Prefix |
Prefix used to name the pictures |
Folder |
Destination folder, must exists |
Number of files |
Number of stored pictures |
Bindable events
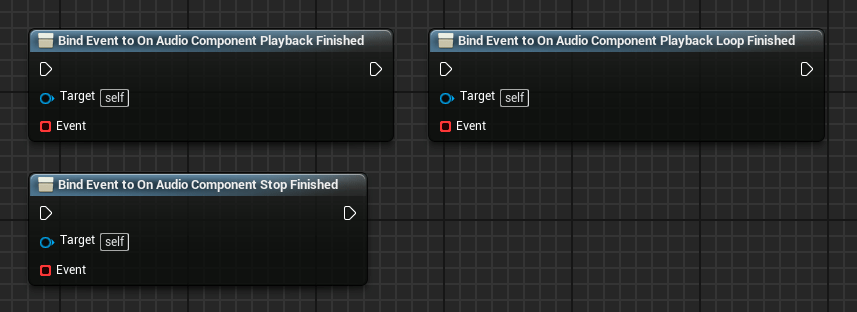
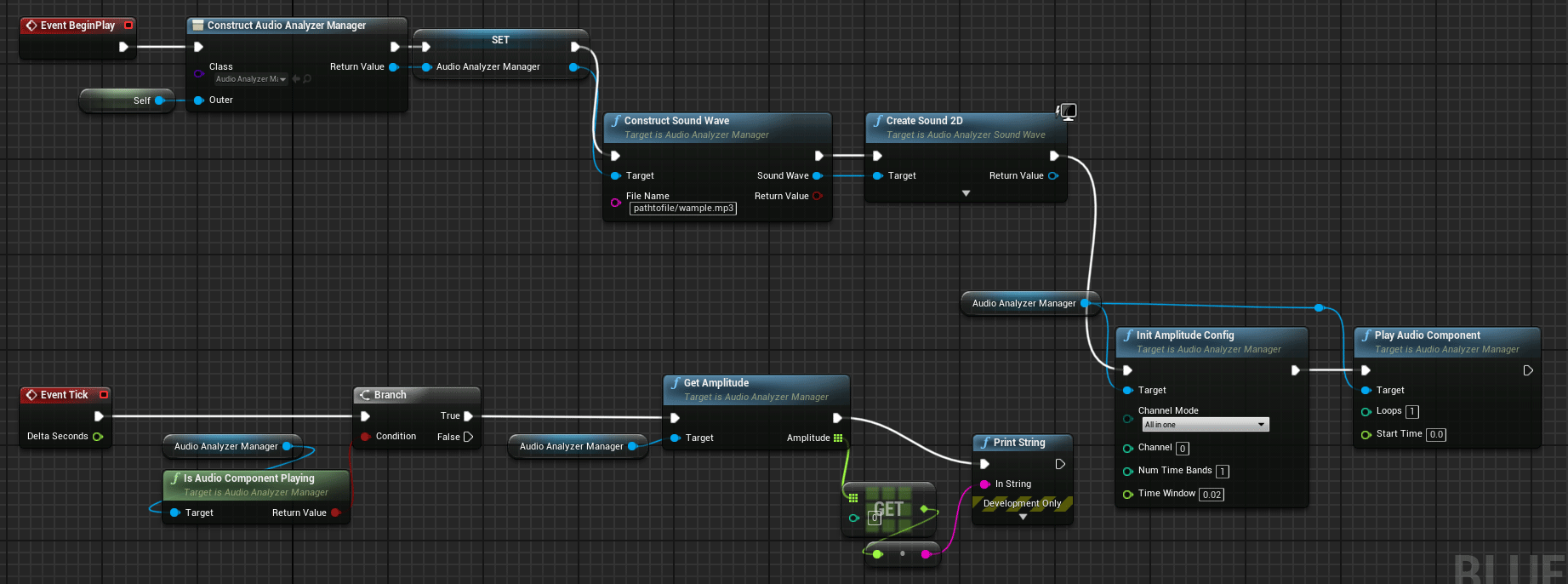
Manager Utils
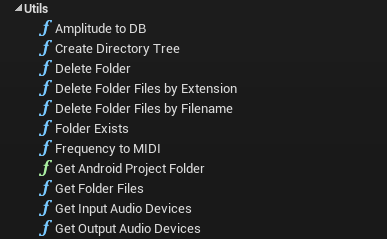
Some extra functions that can be used without an audio source
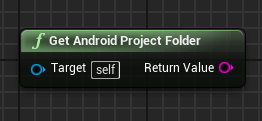
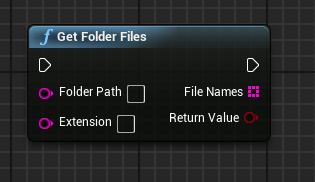
Folder path |
Path to the folder |
Extension |
Extension filter |
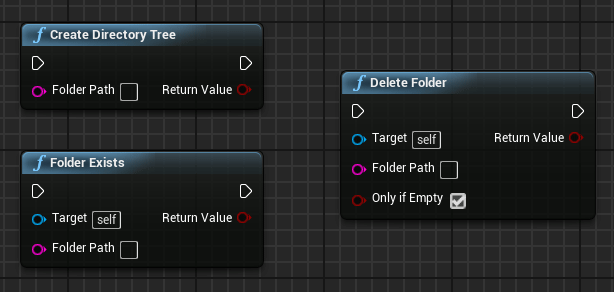
Folder path |
Path to the folder |
Folder path |
Path to the folder |
Folder path |
Path to the folder |
Only If Empty |
Delete the folder only if has no files inside |
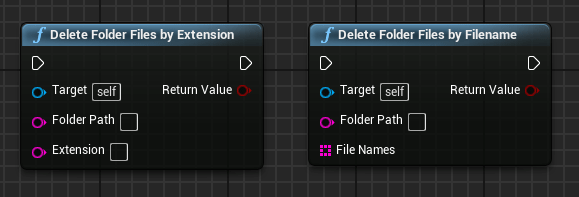
Folder path |
Path to the folder |
Extension |
Extension filter |
Folder path |
Path to the folder |
File Names |
File names container |
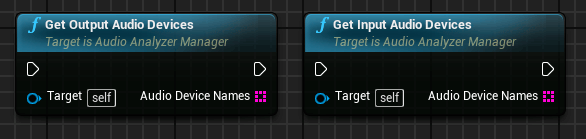
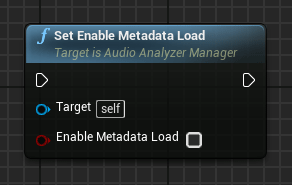
Enable Metadata Load |
Enable metadata information load |
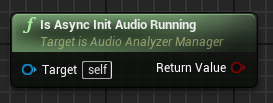
Input nodes flow
Once the audio input source has been initializated any control node can be used to modify the audio playback
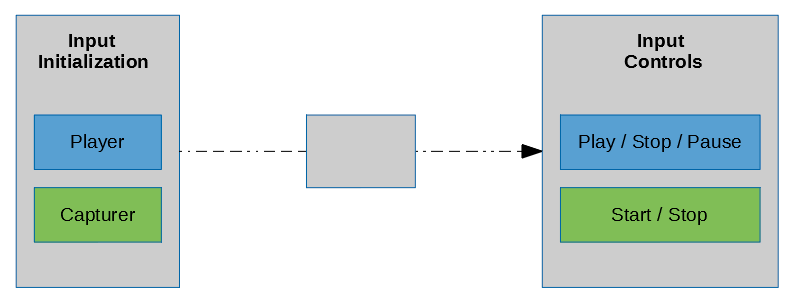