I’m sure that you have seen that check when leaving an application, it is very simple to implement and I will show you how it works
We will use the property isShown() from the class Toast to make a simple exit check to avoid to close de application by a mistake.
Our first step is to add a Toast into our first Activity
private Toast _exitToast;
and make an instance in OnCreate with the message to show, we can use the strings.xml file or directly a string
_exitToast = Toast.makeText(getApplicationContext(), "Push again to exit" , Toast.LENGTH_SHORT);
our last step is to add the override of onKeyDown, we will check is toast is visible in screen to exit with the next back button pulsation
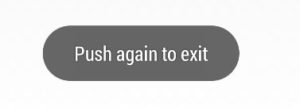
@Override public boolean onKeyDown(int keyCode, KeyEvent event) { if (keyCode == KeyEvent.KEYCODE_BACK && event.getRepeatCount() == 0) { if(_exitToast.getView().isShown()) { //If it is the first activity it will exit _return super.onKeyDown(keyCode, event); } else { _exitToast.show(); } } else return super.onKeyDown(keyCode, event); return false; }
Final full activity code:
package com.parallelcube.example; import android.support.v7.app.ActionBarActivity; import android.os.Bundle; import android.view.KeyEvent; import android.view.Menu; import android.view.MenuItem; import android.widget.Toast; public class MainActivity extends ActionBarActivity { private Toast _exitToast; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); _exitToast = Toast.makeText(getApplicationContext(), "Push again to exit" , Toast.LENGTH_SHORT); } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.main, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId(); if (id == R.id.action_settings) { return true; } return super.onOptionsItemSelected(item); } @Override public boolean onKeyDown(int keyCode, KeyEvent event) { if (keyCode == KeyEvent.KEYCODE_BACK && event.getRepeatCount() == 0) { if(_exitToast.getView().isShown()) { //si esta en el primer estado sale del programa return super.onKeyDown(keyCode, event); } else { _exitToast.show(); } } else return super.onKeyDown(keyCode, event); return false; } }
Now your application can be closed using the double check
Tutorial files
You may also like:
Support this blog!
For the past year I've been dedicating more of my time to the creation of tutorials, mainly about game development. If you think these posts have either helped or inspired you, please consider supporting this blog. Thank you so much for your contribution!