This is the last tutorial of the online leaderboard series. The previous C++ class will be integrated in a blueprint project, with a example to explain how to use the leaderboardManager calls.
Part 1: Server files
Part 2: Client files
Part 3: UE4 game integration
Widgets
Let’s talk about the User Interface, we are going to create two widgets, the first one to show the leaderboard, we can add some buttons to reload the list or reupload the score (the user could have no connection when he got the high score), and some buttons to navigate in the list.
The second widget is used to request info to the user, like the name shown in the list, and show the error messages. This widget can be a child of the first. To control the different states of the widget we can create an enumerator, and set their state before add the widget to the viewport.
We will continue with more blueprint code for this widgets after the preparation the leaderboardManager blueprint. The widget tree looks like this:
- Actor_Game
- UI_Menu_LB
- UI_Menu_LB_info
- UI_Menu_LB
LeaderboardManager Blueprint
Open the game project and create a blueprint class using as parent class our leaderboardManager c++ class.
We are going to override the leaderboardManager functions to interact with the widgets. We can add a reference to the GameBP Actor to simplify the interaction.
When we receive a success sent callback the first thing is to check if the user has canceled the operation during the process to avoid to show the widget. After that we can check if the user has no userId, if the userId is missing that was the first high score sent for this user.
The first sent returns an unique userId, generated in the server files, we need to store it in our savegame to be used in the next game start.
When the score has been sent successfully we can simply report it to the player or we can show the leaderboard with their current position. To do that we can call to the info dialog with a Loading parameter to trigger the leaderboard load process.
When the download of the leaderboard data has been parsed the only thing that we need to do in the blueprint class is to show the leaderboard widget and hide the info dialog.
If we got an error in some request the manager needs to show the info dialog using an Error parameter.
We are going to see more details about this widgets calls in the next sections of the tutorial.
Game Blueprint
Now we can add a variable to the game blueprint using this blueprint type
Before to be able to use the leaderboardManager object we need to initialize it with the Construct Object from Class node.
LoadGameData is an auxiliary function to work with the Savegame class. This function recover the variable values from the last gameplay saved in a physical file. For example, the userId string received in the first score sent must be stored and we need to assign it in the construction of the leaderboardManager too. Each time the player sends a new score this must have the same userId.
When a new record is obtained we need to call the info dialog with the InputName param to show a dialog with an input field, this field can be used to implement multiple checks over the name, like maximum/minimum length or limit the use of inappropriate words
The other important call is the leaderboard button, to request our top 100 list we only need to call the info dialog with the Loading parameter.
Leaderboard-widget
When we call to show the leaderboard widget the manager has already received all the data of the top 100 list, we only need to extract the data from the manager and put it in the textblock of the widget. The UpdateData funtion will prepare the variables of the widget like how much tabs must be contain, or if the player is in the top, navigates to show the current tab for his position. The LoadLeaderboard takes the data for the current tab and fill each column, we could apply some list effects in this function, like an incremental row appearance.
The buttons to navigate in the list just update the related variables an do a call to LoadLeaderboard. This calls don’t request for new data to the server, just extract it from the manager data.
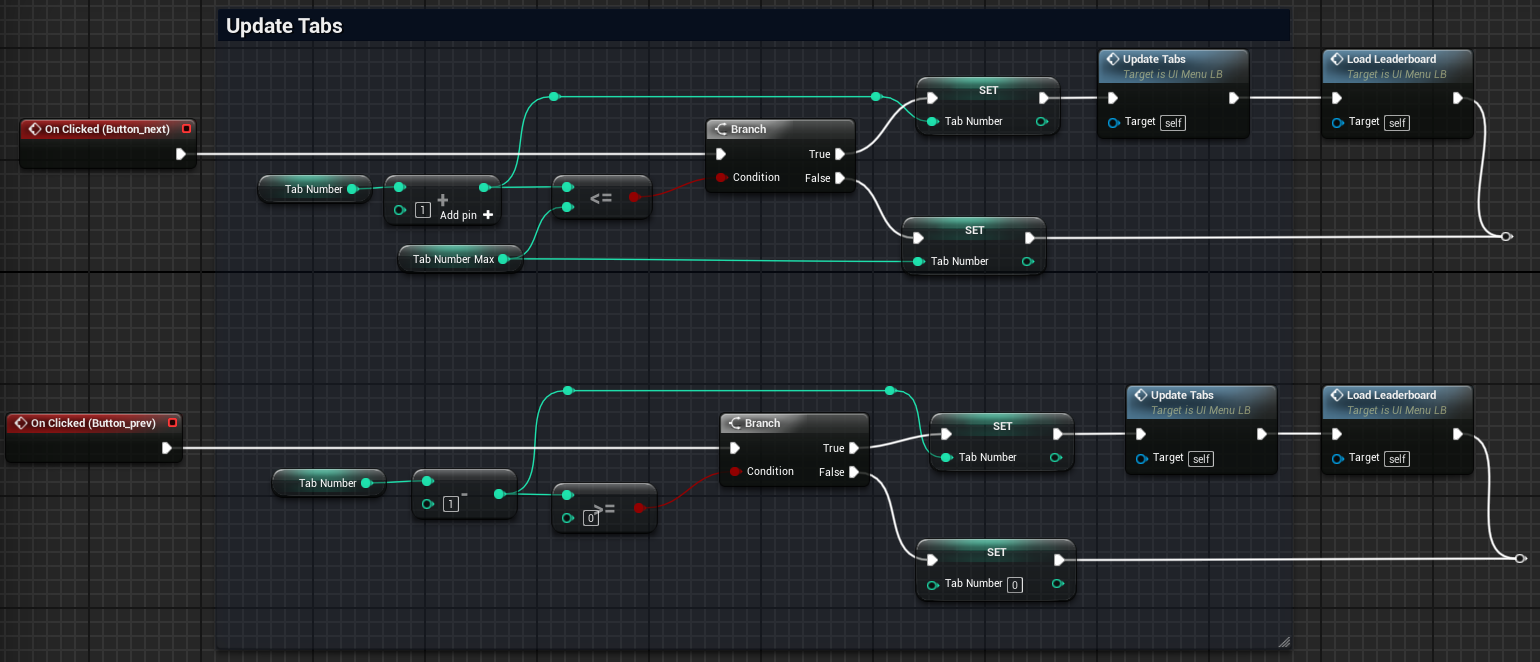
Finally the reload button do a call to the info dialog to request a new top 100 list, and the reupload button has the same behavior than a new record, shows the input name dialog to trigger the request process.
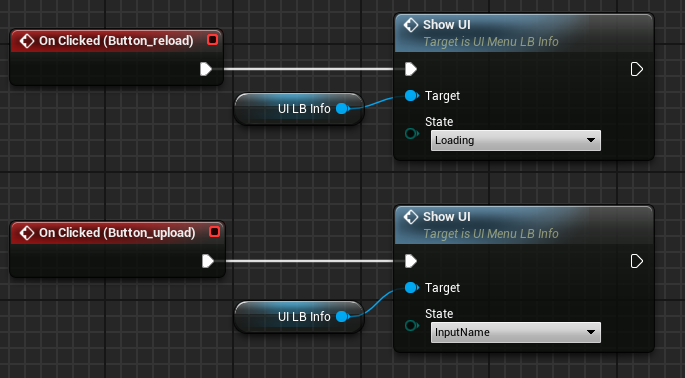
Now we can see in depth the UpdateData function. The first part calculates the widget variables like the tab limits and the current player tab.
The middle is only to hide the player row if he has no high score yet
The last part is to fill de textblocks with the player best score
The LoadLeaderboard function iterates for the current tab elements
In each iteration it append the data for one row to each column (rank, score, name)
Finally we set the text of the textblocks for each column with the variables updated during the loop.
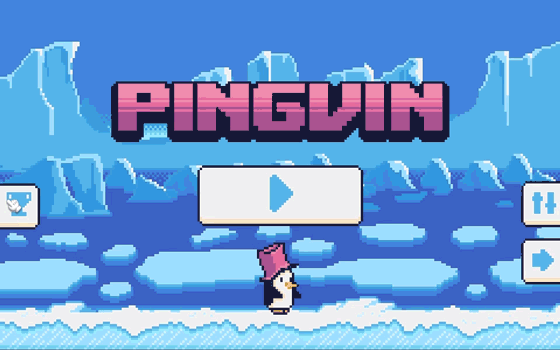
Leaderboard-info-widget
The info dialog is used like a trigger, when is called with a Loading param it triggers the getLeaderboard request , on the other hand, if is called with a Sending param it triggers the SendScore request of our leaderboard manager.
The UpdateData function for this widget just controls the text and visibility for each component depending on the dialog state.
The action executed by the dialog button depends on the state too. For the InputName state the button must be the trigger for the sending process, otherwise it is used as a cancel button of the current request.
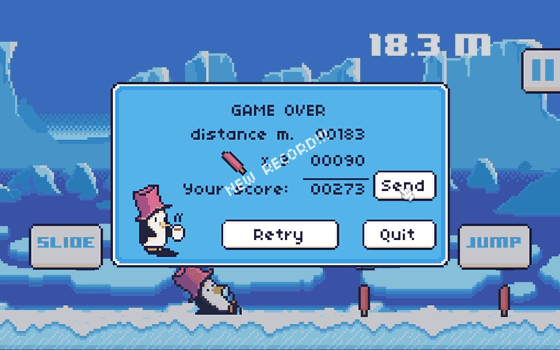
All we need to do now is add some animation effects on buttons and texts to do the leaderboard more visually attractive, don’t forget to add some sounds too š
Now our game has a light leaderboard online system with witch players can accept the challenge of getting the first ranking positions
Tutorial files
2021/10/05 – Updated to PHP 7 and Unreal Engine 4.27
You may also like:
Support this blog!
For the past year we have been dedicating more of our time to the creation of tutorials, mainly about game development. If you think these posts have either helped or inspired you, please consider supporting this blog. Thank you so much for your contribution!